注:本文已发布超过一年,请注意您所使用工具的相关版本是否适用
序
笔记
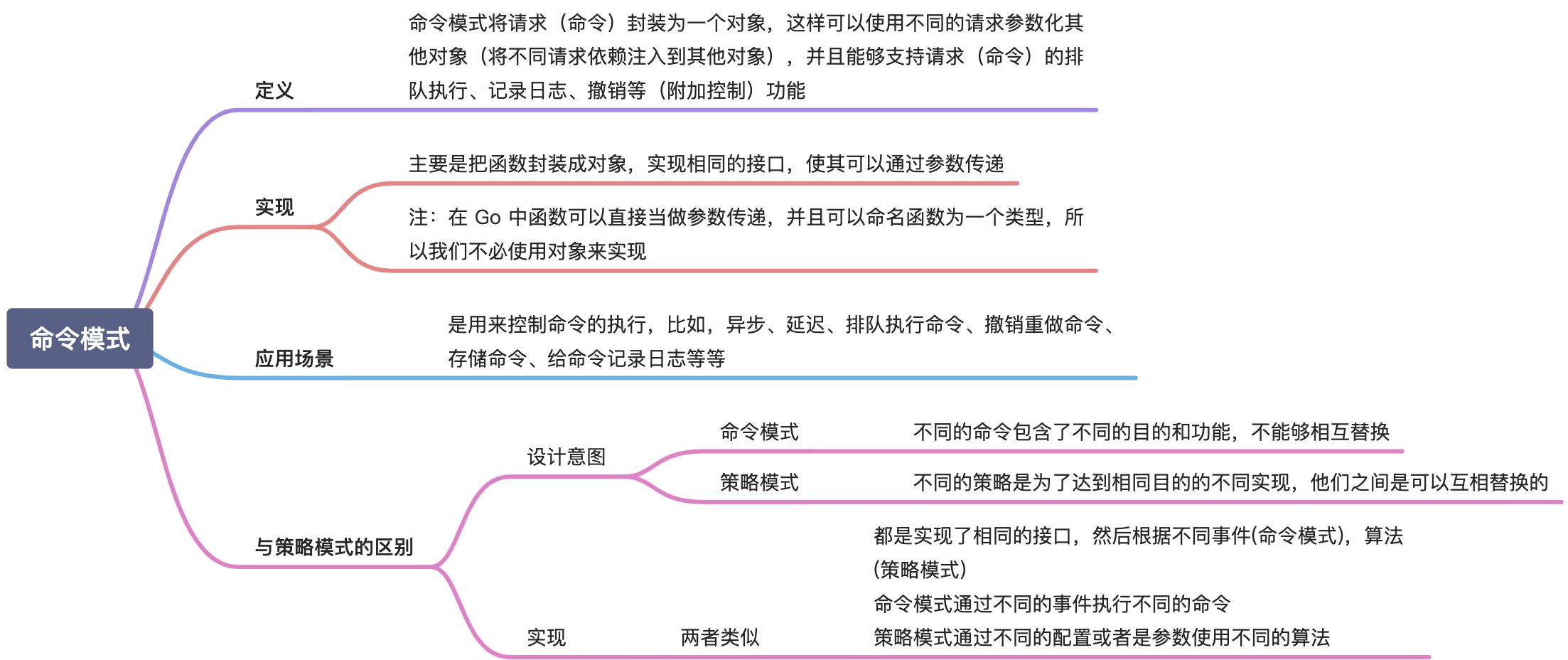
代码实现
接下来会有两个例子,第一个是按照原文定义的方式,将函数封装成对象,第二个例子我们直接将函数作为参数传递。
将函数封装为对象
Code
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43
|
package command
import "fmt"
type ICommand interface { Execute() error }
type StartCommand struct{}
func NewStartCommand( /*正常情况下这里会有一些参数*/ ) *StartCommand { return &StartCommand{} }
func (c *StartCommand) Execute() error { fmt.Println("game start") return nil }
type ArchiveCommand struct{}
func NewArchiveCommand( /*正常情况下这里会有一些参数*/ ) *ArchiveCommand { return &ArchiveCommand{} }
func (c *ArchiveCommand) Execute() error { fmt.Println("game archive") return nil }
|
测试
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54
| package command
import ( "fmt" "testing" "time" )
func TestDemo(t *testing.T) { eventChan := make(chan string) go func() { events := []string{"start", "archive", "start", "archive", "start", "start"} for _, e := range events { eventChan <- e } }() defer close(eventChan)
commands := make(chan ICommand, 1000) defer close(commands)
go func() { for { event, ok := <-eventChan if !ok { return }
var command ICommand switch event { case "start": command = NewStartCommand() case "archive": command = NewArchiveCommand() }
commands <- command } }()
for { select { case c := <-commands: c.Execute() case <-time.After(1 * time.Second): fmt.Println("timeout 1s") return } } }
|
将函数直接作为参数
Code
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31
|
package command
import "fmt"
type Command func() error
func StartCommandFunc() Command { return func() error { fmt.Println("game start") return nil } }
func ArchiveCommandFunc() Command { return func() error { fmt.Println("game archive") return nil } }
|
测试
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55
| package command
import ( "fmt" "testing" "time" )
func TestDemoFunc(t *testing.T) { eventChan := make(chan string) go func() { events := []string{"start", "archive", "start", "archive", "start", "start"} for _, e := range events { eventChan <- e }
}() defer close(eventChan)
commands := make(chan Command, 1000) defer close(commands)
go func() { for { event, ok := <-eventChan if !ok { return }
var command Command switch event { case "start": command = StartCommandFunc() case "archive": command = ArchiveCommandFunc() }
commands <- command } }()
for { select { case c := <-commands: c() case <-time.After(1 * time.Second): fmt.Println("timeout 1s") return } } }
|
关注我获取更新
猜你喜欢